Adding Vanilla JavaScript UI Components to a Vue.js App Using a templateRef
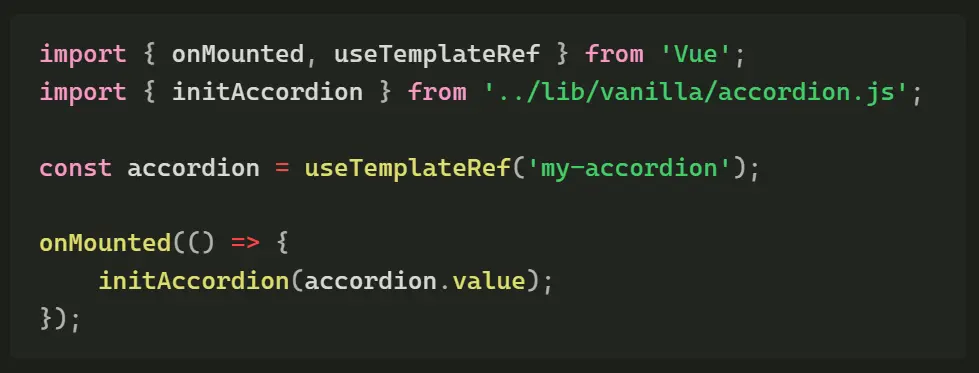
One of my favorite use cases of JavaScript application libraries such as Vue.js is to add them to a single page of an otherwise normal website. Occasionally, you need some extra special functionality on a particular page, and rolling up a small Vue app to only load on this one page is a great solution. However, you may already have a number of JavaScript components built for your UI which your one off Vue application may also need. In your Vue components you can use a reference to the necessary DOM element to initialize the vanilla JavaScript functionality.
Enter the Accordion
For this example, we assume that we need to implement an accordion (a.k.a. a disclosure widget, a.k.a. a drawer, a.k.a. a collapse) in our Vue application. This UI element is used throughout the site, and now we need it in our Vue app.
Note that this example is using Vue 3 with the Composition API.
Normally, when I make a JS module I include an init
function that takes as an argument the DOM element that I'm enhancing. In this case, we have a function like this:
initAccordion(el) {// make an accordion out of el}
Create the Accordion Ref
Vue handles the creation and manipulation of our DOM elements, and we don't normally control how and when they are created, updated, or destroyed. With the useTemplateRef
function we can obtain a reference to an actual DOM element created by Vue and manipulate it as we normally would.
In our accordion getting a reference to the accordion element looks like this:
<script setup>import { useTemplateRef } from 'Vue';const accordion = useTemplateRef('my-accordion');</script><template><details ref="my-accordion"><summary>The Accordion</summary><div class="accordion-body"></div></details></template>
First, we import the useTemplateRef
from Vue. Then we declare a variable accordion
which is set to the reference created by useTemplateRef
. We pass a string identifier to the function, which we also set as the value of the ref
attribute of our accordion element in the template section of the component.
Now accordion.value
will point to the DOM element of our accordion.
Read the documentation for useTemplateRef
Implement the Accordion Functionality.
I realize that the details
element can handle the opening and closing of our accordion by default, but for the sake of our example let's assume that we're adding additional functionality.
We need to make sure our Vue component is finished rendering, so we initialize our accordion inside of the onMounted
lifecycle hook. This ensures that our accordion element actually exists in the DOM and is ready to go.
The script
section of our Vue component now looks like this:
import { onMounted, useTemplateRef } from 'Vue';import { initAccordion } from '../lib/vanilla/accordion.js';const accordion = useTemplateRef('my-accordion');onMounted(() => {initAccordion(accordion.value);});
Again we start by importing the necessary scripts. We add Vue's onMounted
hook and an import for initAccordion
—the implementation function for our accordion from our vanilla JS modules. For this example, we assume our accordion.js file is in a lib/vanilla
directory one level above our component.
Then inside of onMounted
we call initAccordion
passing the reference to
our accordion DOM element, accordion.value
.
Now, whenever our Vue component is mounted, the accordion element will be turned in to a functional UI module using existing our vanilla JS.
Why Not Implement the Accordion as a Vue Component?
You could do this, and if your entire application was in Vue I would recommend doing just that. In our use case, however, we are building a small Vue app to insert into a larger project where the UI functionality has already been defined in vanilla JS. Under these circumstances, it is more efficient to define the functionality once for use both inside and outside of Vue. It saves time and saves you from having to make updates to the JS functionality in two places.